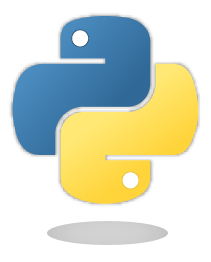
(1) sort 方法:sort方法是將串列元素從小至大排序,語法如下:
串列變數名稱.sort(reverse=False)
說明:參數中reverse預設為False,可不寫,功能為由小至大排序,如果reverse設為True,則為由大至小排序
範例1:將分數由小至大排序
score = [89,76,68,88,59,100,47,96]
score.sort()
執行結果:score 的值為 [47, 59, 68, 76, 88, 89, 96, 100]
範例2:將分數由大至小排序
score = [89,76,68,88,59,100,47,96]
score.sort(reverse=True)
執行結果:score 的值為 [100, 96, 89, 88, 76, 68, 59, 47]
(2) sorted方法:sorted方法與sort方法的差異在於sort方法會變更原來變數的值,而sorted方法在執行過後不會變更原來變數值,所以要將結果存至另一變數,語法如下:
串列變數2=串列變數1.sorted(reverse=False)
說明:將串列變數1排序後的值存至串列變數2,參數中reverse預設為False,可不寫,功能為由小至大排序,如果reverse設為True,則為由大至小排序
範例3:將分數由小至大排序並且存至另一變數
score1 = [89,76,68,88,59,100,47,96]
score2 = sorted(score1)
執行結果:score1 的值為 [89,76,68,88,59,100,47,96]
score2 的值為 [47, 59, 68, 76, 88, 89, 96, 100]
範例4:將分數由大至小排序並且存至另一變數
score1 = [89,76,68,88,59,100,47,96]
score2 = sorted(score1,reverse=False)
執行結果:score1 的值為 [89,76,68,88,59,100,47,96]
score2 的值為 [100, 96, 89, 88, 76, 68, 59, 47]
(3) reverse方法:由字義可知此方法會將串列反轉排序,有鏡射的意思,語法如下:
串列變數名稱.reverse()
範例5:將字串串列反轉排序
week = ['星期日','星期一', '星期二','星期三','星期四','星期五', '星期六']
week.reverse()
執行結果:week 的值為 ['星期六', '星期五', '星期四', '星期三', '星期二', '星期一', '星期日']
完整程式碼如下:
- # -*- coding: utf-8 -*-
- """
- Created on Fri Jan 4 11:39:05 2019
- @author: 軟體罐頭
- """
- print('範例 1:將分數由小至大排序')
- score = [89,76,68,88,59,100,47,96]
- print('score 排序前 = ',score)
- score.sort()
- print('score 排序後 = ',score)
- print()
- print('範例 2:將分數由大至小排序')
- score = [89,76,68,88,59,100,47,96]
- print('score 排序前 = ',score)
- score.sort(reverse=True)
- print('score 排序後 = ',score)
- print()
- print('範例3:將分數由小至大排序並且存至另一變數')
- score1 = [89,76,68,88,59,100,47,96]
- print('score1 排序前 = ',score1)
- score2 = sorted(score1)
- print('score1 排序後 = ',score1)
- print('score2 排序後 = ',score2)
- print()
- print('範例4:將分數由大至小排序並且存至另一變數')
- score1 = [89,76,68,88,59,100,47,96]
- print('score1 排序前 = ',score1)
- score2 = sorted(score1,reverse=True)
- print('score1 排序後 = ',score1)
- print('score2 排序後 = ',score2)
- print()
- print('範例5:將字串串列反轉排序')
- week = ['星期日','星期一', '星期二','星期三','星期四','星期五', '星期六']
- print('week 排序前 = ',week)
- week.reverse()
- print('week 排序後 = ',week)
執行結果如下: